Tips & tricks you might want to use on your project
A javascript library to display a coverflow effect - coverflowjs
Coverflow is an alternative to a carousel for displaying a series of images. Click here to see an example.
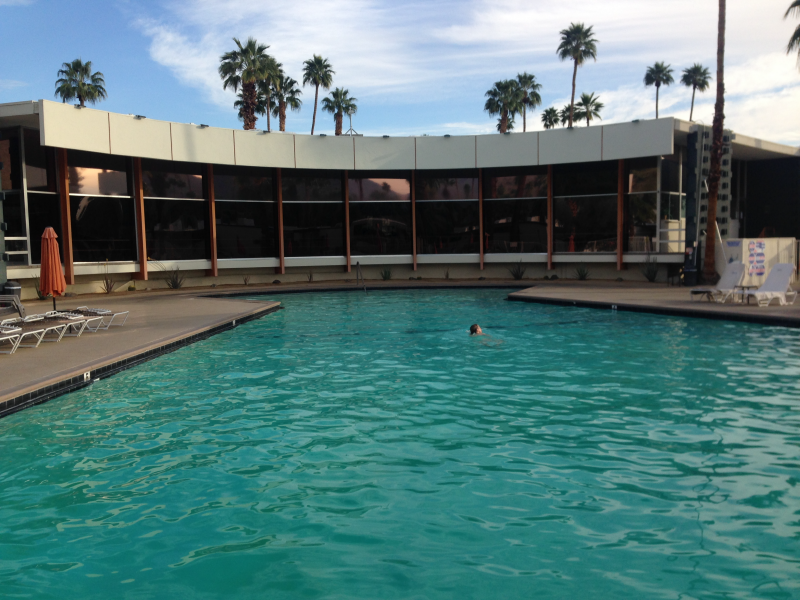
Our resort's swimming pool
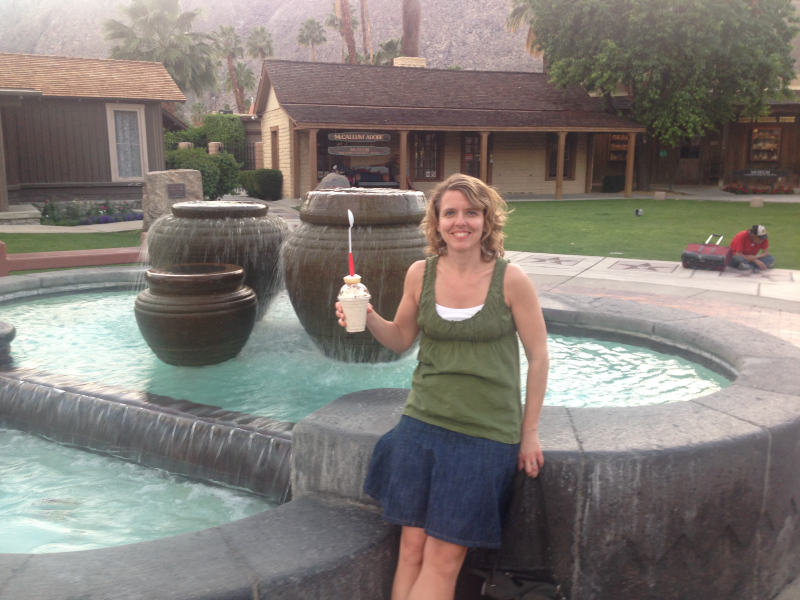
A Maple pan-shake!
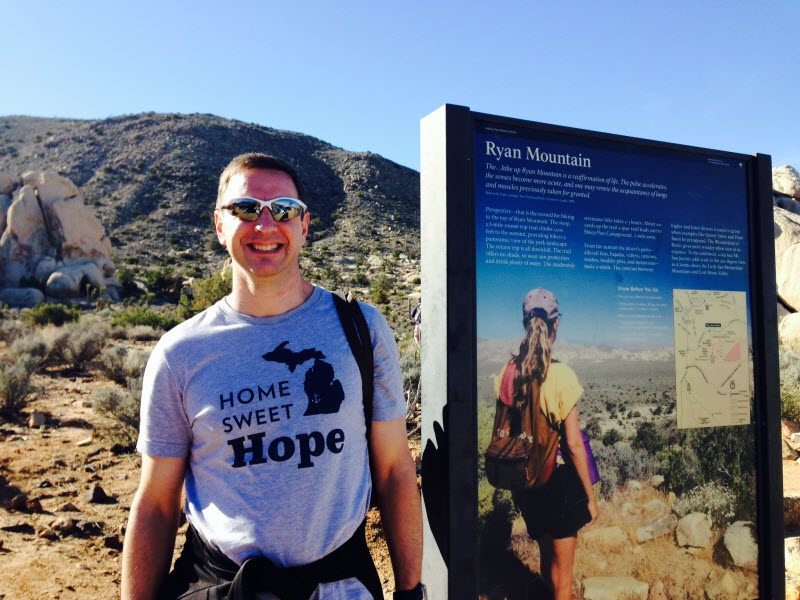
At the base of Ryan Mountain
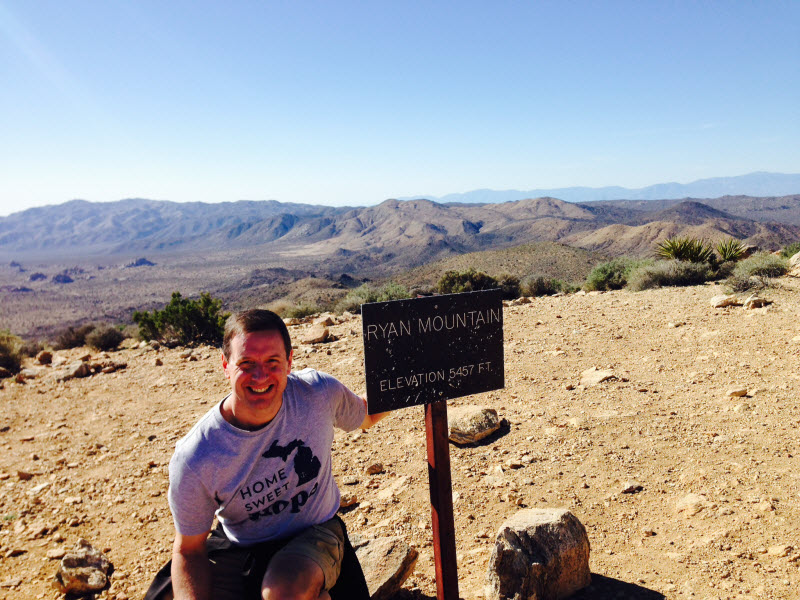
At the top of Ryan Mountain!
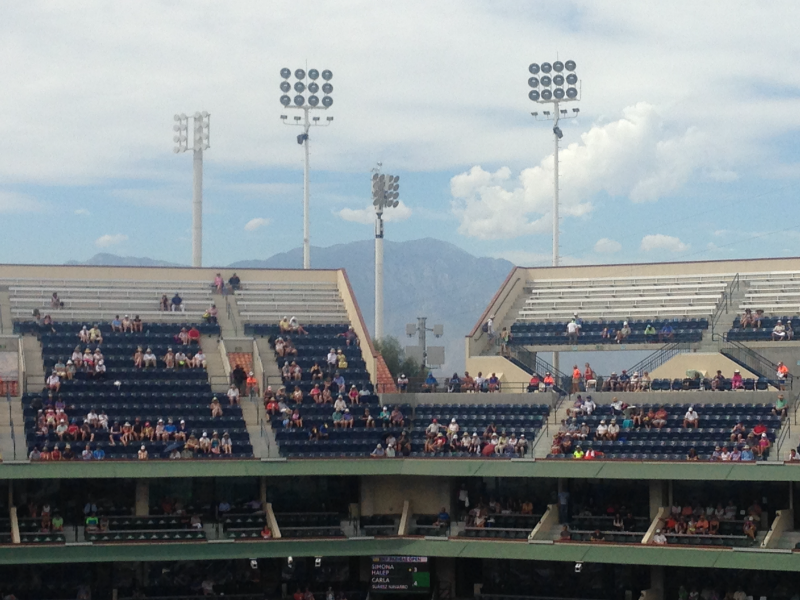
The tennis stadium with mountains in the background
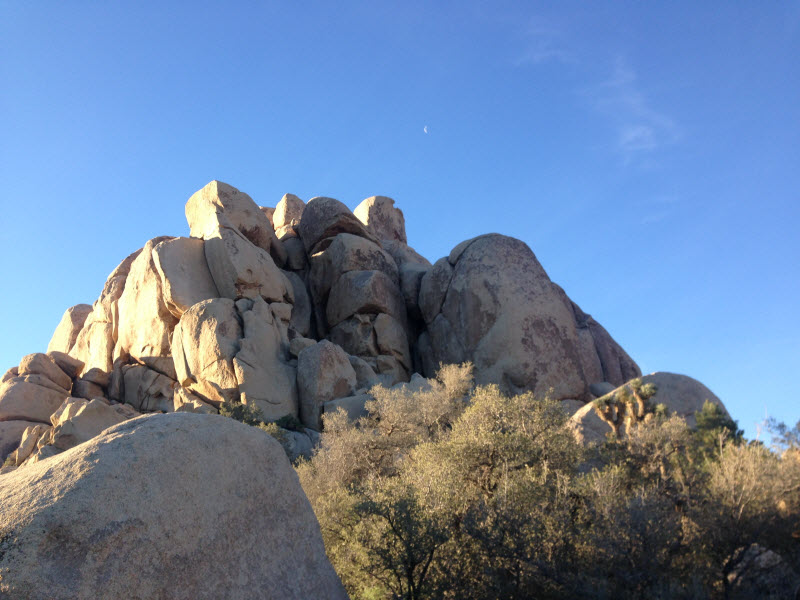
An interesting rock formation
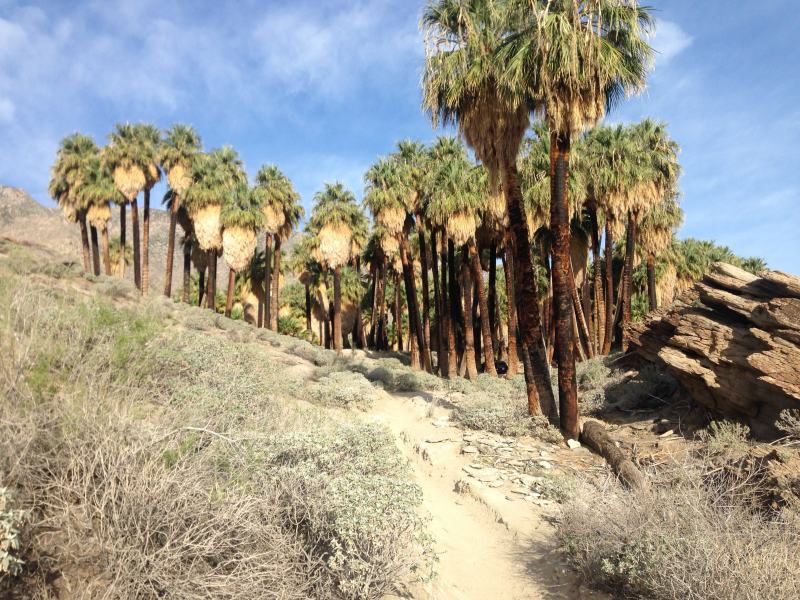
Some palm trees in Indian Canyons
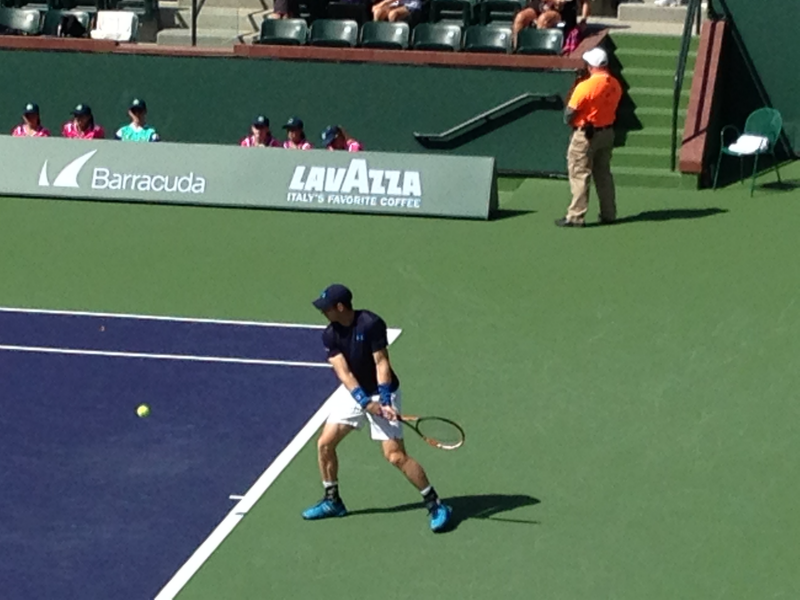
Andy Murray hitting a backhand
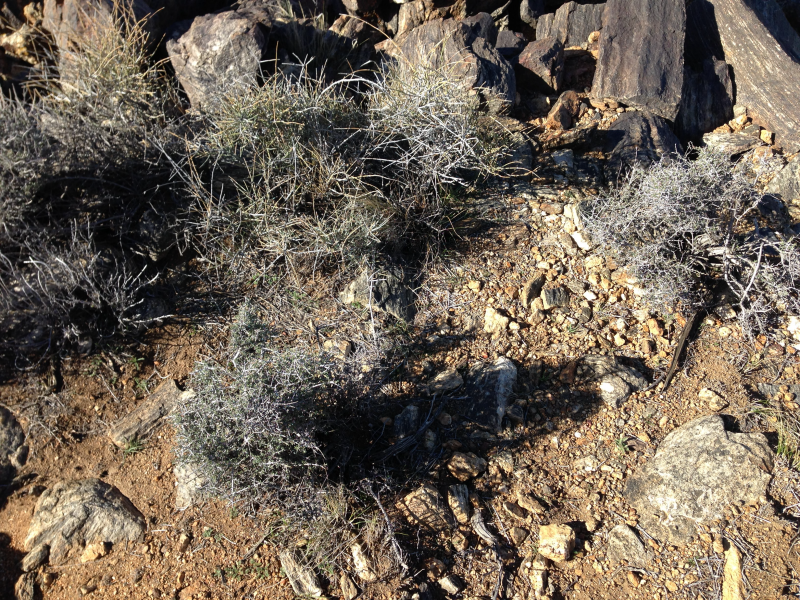
An interesting cactus plant
The coverflowjs library makes creating a coverflow effect fairly simple. To use the library you will need to do the following while logged on to your remote desktop:
- Download the zip file containing the coverflow CSS and JavaScript files from
- Open the Zip file and navigate into the folder coverflow-3.0.1dist
- Copy the files coverflow.min.js and coverflow.css into the site root folder for your site
- Attach the stylesheet coverflow.css to your page or template
- Add a script element whose src attribute references the coverflow.js library into the desired page or template, before the document's body ending tag.
You'll need to have the standard Javascript references to JQuery and Bootstrap's Javascript from the Moodle page URLs needed to use Bootstrap in your document before this reference.
Setting up the HTML structure
Add HTML similar to this into your page:
<div id="coverflow-wrapper"> <div id="coverflow"> <img src="images/image1.jpg"/> <img src="images/image2.jpg"/> <img src="images/image3.jpg"/> </div> </div>
Each element within the div id="coverflow" will be one element of the displayed coverflow — it can, for example, be a div containing an img, if you want to add captions to the pictures (this is what was done in the example above).
Note that some experimenting does indicate that the pictures (or other elements) making up the coverflow must be the same size in order for the effect to work correctly.
Initialization
The steps needed to initialize the coverflow component differ depending on whether you're using AngularJS within your web site.
Are you using Angular JS?
Initialization the coverflow component without AngularJS
Add the following initialization Javascript code to the page or template, right before the end tag for the body element:
<script> $(window).load(function() { $('#coverflow').coverflow(); }); </script>
The #coverflow
between the single quotes is a CSS selector, and should match the element that contains the elements making up the coverflow effect.
With the above initialization code, the first element in the coverflow will be the "active" one. To change this, modify the above initialization code as shown below:
<script> $(window).load(function() { $('#coverflow').coverflow({active: 2}); }); </script>
where 2 indicates that the 3rd element will be active, since as with carousels, the coverflow Javascript code starts counting at 0.
If you want to get really fancy, this version of the initialization code will automatically make the middle element active, regardless of how many there are:
<script> $(window).load(function() { $('#coverflow').coverflow({ active: Math.floor($('#coverflow').children().length/2) }); }); </script>
Initialization of the coverflow component when using AngularJS
Complete the following steps to make the coverflow component work in a site using AngularJS.
- Add an attribute named data-coverflow to the div element containing the img elements, so that it looks like this:
<div id="coverflow-wrapper"> <div id="coverflow" data-coverflow> <img src="images/image1.jpg"/> <img src="images/image2.jpg"/> <img src="images/image3.jpg"/> </div> </div>
You can optionally give a value to the data-coverflow attribute to indicate which of the img elements should be the one displayed as the "highlighted" image initially. - Add script element with the src value of http://scripts.hopewebdesign.net/150-coverflow.js after an existing script elements in your index.html file.
-
Modify the declaration of your Angular module (likely in routing.js if you are starting from your Lab 10 files) so that it adds the
150-coverflow module as an additional dependency. It will look something like this:
var app = angular.module("lab10", ['ngRoute', '150-coverflow']);
with the new code shown in bold.
A CSS note
The coverflowjs library uses absolute positioning to implement the coverflow effect. This can have the unfortunate effect of causing text under the coverflow element to overlap the coverflow. To solve this in my example above, I added the following CSS rules
.ui-coverflow-wrapper { height: 225px; }
The coverflowjs library adds the class .ui-cover-flow-wrapper to the parent of the div containing the images. Since absolutely positioned content doesn't have any height by default, it's necessary to set an explicit height for that element.
The 225px value specified above is based on the height of the images in the coverflow; you should use an appropriate value for your images.